Sometimes it is really helpful to use debug mode to see what really happens during runtime of your code. And that is the time for delve.go code debugger.
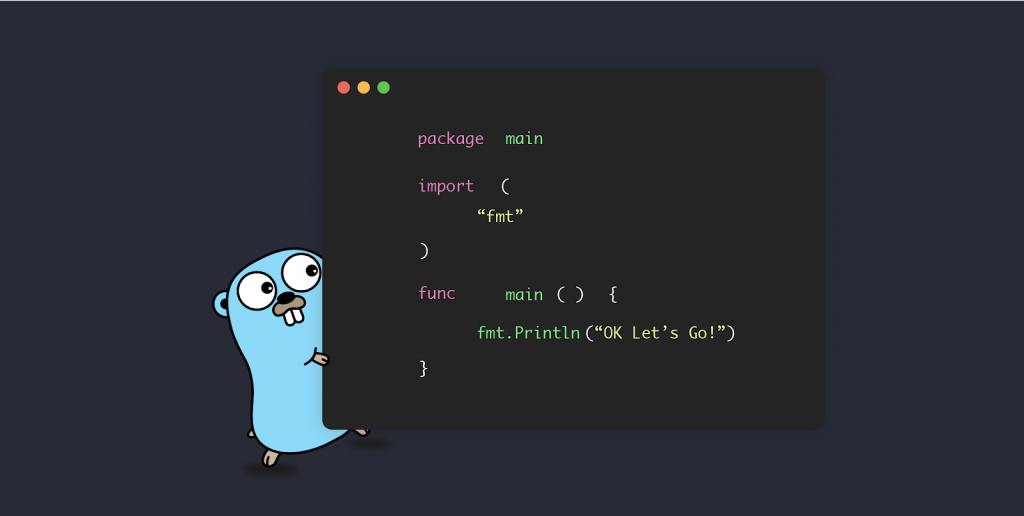
How to install delve (if you have your go path set)
go get -u github.com/derekparker/delve/cmd/dlv
Now you should be able to run the dlv version
dlv version
Delve Debugger
Version: 1.4.0
Build: $Id: 1990ba12450cab9425a2ae62e6ab988725023d5c $
I have prepared this simple go code
type Person struct {
Name string
Age int
}
func main(){
var me Person
me.Name = "Andrew"
me.Age = 19
var person2 Person
person2.Name = "Andy"
person2.Age = 10
var person3 Person
person3.Name = "Paul"
person3.Age = 20
fmt.Println(me)
}
Now let’s start debugging with delve
dlv debug delve.go
Now you press ‘h’ to list help menu, which is really useful to set breakpoint, type break (shortcut is ‘b’) delve.go:20 This command will set breakpoint on line 20 in delve.go To run the debug, just type ‘continue’ and it will stop at the first breakpoint
(dlv) continue
> main.main() ./delve.go:20 (hits goroutine(1):1 total:1) (PC: 0x49b3d6)
15: me.Name = "Andrew"
16: me.Age = 19
17:
18: var person2 Person
19: person2.Name = "Andy"
=> 20: person2.Age = 10
21:
22: var person3 Person
23: person3.Name = "Paul"
24: person3.Age = 20
Here we can see that the breakpoint is set on line 20, if I wanted to see the value of Person2 object, I can simply write ‘print person2’, the output is as following:
(dlv) print person2
main.Person {Name: "Andy", Age: 0}
Or we can print each property of the object, for example name of person2
(dlv) print person2.Name
"Andy"